Computing Fibonacci numbers with Linear Algebra
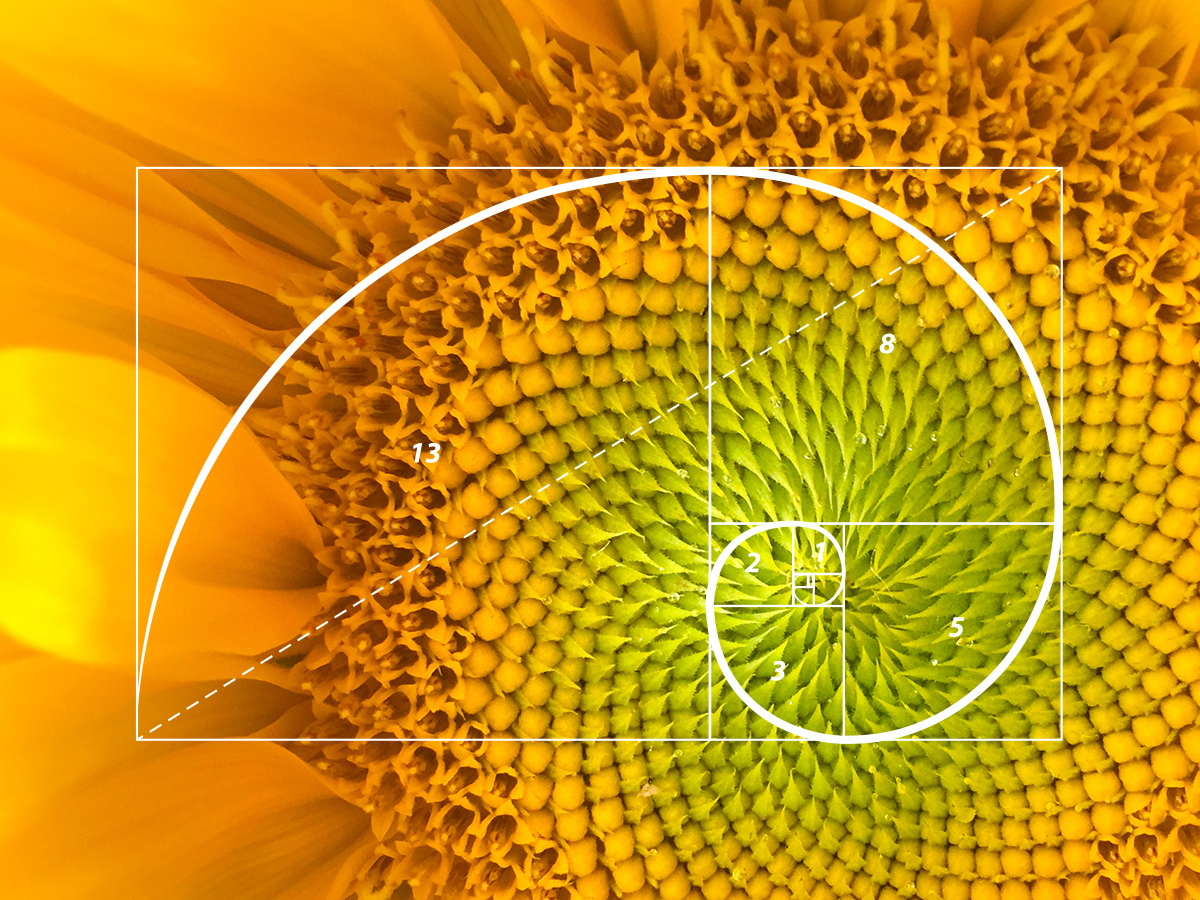
The Fibonacci numbers are a sequence of numbers where every new entry is the sum of the previous two:
\[0, 1, 1, 2, 3, 5, 8, 13, ...\]
To solidify the rule for this sequence mathematically, we can write the equation for the next Fibonacci number as the sum of the two previous entries in the sequence:
\[F_{k+2} = F_{k+1} + F_{k}\]
We begin by putting the previous and next previous entries into a vector \(u_k\):
\[u_k = \begin{bmatrix} F_{k+1}\\ F_{k} \end{bmatrix}\]
This vector \(u_k\) represents our current state, notice that the Fibonacci number of interest \(F_k\) is the second entry in this vector. To find the next state vector \(u_{k+1}\), we need to apply a transformation to the current state vector \(u_k\) which represents a single step forward in the sequence. We do so by multiplying the current state vector by a matrix \(A\) which is defined as follows:
\[A = \begin{bmatrix} 1 & 1\\ 1 & 0 \end{bmatrix}\]
Since multiplication by \(A\) represents a single step forward in the sequence, it's clear that we can start from \(u_0 = (1, 0)\) which represents the initial state vector and multiply by \(A\) one-hundred times to find \(u_{100}\):
\[u_{100} = A^{100}u_0\]
That's great, but \(A\) is a non-diagonal matrix so calculating \(A^{100}\) isn't as straight-forward as raising each element to the hundredth power. Instead, we need to diagonalize the matrix by finding the eigenvalues and eigenvectors of \(A\) and factorizing it into the form \(X \Lambda X^{-1}\). Here, \(X\) represents the eigenvector matrix and \(\Lambda\) represents the diagonal eigenvalue matrix. You might be asking what this factorization does for us. It just so happens that this form makes finding matrix powers almost trivial to compute.
Consider again the matrix power \(A^{100}\), then rewrite the equation as \((X \Lambda X^{-1})^{100}\). One of the properties of this factorization is that raising it to any power \(k\) is the same as raising only the eigenvalue matrix to the power \(k\):
\[A^{k} = (X \Lambda X^{-1})^{k}\ = X \Lambda X^{-1} X \Lambda X^{-1} ... X \Lambda X^{-1}\]
The pairs of \(X^{-1} X\) become the identity matrix, and we are left with:
\[A^{k} = X \Lambda^{k} X^{-1}\]
Since we only need to raise the eigenvalue matrix to the power \(k\), the problem has become much easier. The matrix power of a diagonal matrix is as simple as raising each element to that power. So, the next step is to actually find the eigenvalues and eigenvectors of \(A\), then we will have \(X\) and \(\Lambda\), and be able to derive \(X^{-1}\).
Finding the eigenvalues and eigenvectors
To find the eigenvalues of \(A\), we solve for \(\lambda\) in the equation \(\begin{vmatrix} A - \lambda I \end{vmatrix} = \begin{vmatrix} 1 - \lambda & 1\\ 1 & -\lambda \end{vmatrix} = 0\)
\[\begin{vmatrix} A - \lambda I \end{vmatrix} = (1 - \lambda)(-\lambda) - (1)(1) = 0\]
\[\lambda^{2} - \lambda - 1 = 0\]
Using the quadratic formula, we find that \(\lambda = \frac{1 \pm \sqrt{5}}{2}\). Thus, \(\lambda_{1} = \frac{1 + \sqrt{5}}{2}\) and \(\lambda_{2} = \frac{1 - \sqrt{5}}{2}\)
Now we need to find the corresponding eigenvectors \(x_{1}\) and \(x_{2}\). We need to answer the question, for what vector \(x\) does \((A - \lambda I)x = 0\)? We know that for \(\lambda_{1}\) and \(\lambda_{2}\), the following equation holds: \(\lambda^{2} - \lambda - 1 = 0\).
If \(x = \begin{bmatrix} a \\ b \end{bmatrix}\), then distributing \(x\) in \((A - \lambda I)x = 0\) yields \(a(1 - \lambda) + b = 0\) which can be rewritten as \(a\lambda - a - b = 0\). This looks familiar, let's set \(a = \lambda\) and \(b = 1\) to arrive at \(\lambda^{2} - \lambda - 1 = 0\). We conclude that for \(\lambda_{1}\) the corresponding eigenvector is \(\begin{bmatrix} \lambda_{1} \\ 1 \end{bmatrix}\) and for \(\lambda_{2}\) the corresponding eigenvector is \(\begin{bmatrix} \lambda_{2} \\ 1 \end{bmatrix}\)
Finally, we have all the pieces \(\lambda_{1}\), \(\lambda_{2}\), \(x_{1}\), and \(x_{2}\)
Diagonalizing \(A\) as \(X \Lambda X^{-1}\)
With \(\lambda_{1}\), \(\lambda_{2}\), \(x_{1}\), \(x_{2}\) found, we can represent the eigenvector matrix and eigenvalue matrix as follows:
\[X = \begin{bmatrix} x_{1} & x_{2} \end{bmatrix} = \begin{bmatrix} \lambda_{1} & \lambda_{2} \\ 1 & 1 \end{bmatrix}\]
\[\Lambda = \begin{bmatrix} \lambda_{1} & 0 \\ 0 & \lambda_{2} \end{bmatrix}\]
Then, we need to compute the inverse eigenvector matrix \(X^{-1}\):
\[ X^{-1} = \frac{1}{\begin{vmatrix} X \end{vmatrix}} \begin{bmatrix} 1 & -\lambda_{2} \\ -1 & \lambda_{1} \end{bmatrix} = \frac{1}{\lambda_{1} - \lambda_{2}} \begin{bmatrix} 1 & -\lambda_{2} \\ -1 & \lambda_{1} \end{bmatrix} \]
And the matrix power of the eigenvalue matrix \(\Lambda^{k}\):
\[ \Lambda^{k} = \begin{bmatrix} \lambda_{1}^{k} & 0 \\ 0 & \lambda_{2}^k \end{bmatrix} \]
Finally, let's solve \(A^{k} = X \Lambda^{k} X^{-1}\):
\[ A^{k} = X \Lambda^{k} X^{-1} = \frac{1}{\lambda_{1} - \lambda_{2}} \begin{bmatrix} \lambda_{1} & \lambda_{2} \\ 1 & 1 \end{bmatrix} \begin{bmatrix} \lambda_{1}^{k} & 0 \\ 0 & \lambda_{2}^{k} \end{bmatrix} \begin{bmatrix} 1 & -\lambda_{2} \\ -1 & \lambda_{1} \end{bmatrix} \]
Solving \(u_{k} = A^{k}u_{0}\)
With \(A^{k}\) diagonalized as \(X \Lambda^{k} X^{-1}\), we find \(u_k\) which is the state vector at "time" k:
\[ u_{k} = A^{k}u_{0} = X \Lambda^{k} X^{-1} u_{0} \]
\[ u_{k} = \frac{1}{\lambda_{1} - \lambda_{2}} \begin{bmatrix} \lambda_{1} & \lambda_{2} \\ 1 & 1 \end{bmatrix} \begin{bmatrix} \lambda_{1}^{k} & 0 \\ 0 & \lambda_{2}^{k} \end{bmatrix} \begin{bmatrix} 1 & -\lambda_{2} \\ -1 & \lambda_{1} \end{bmatrix} \begin{bmatrix} 1 \\ 0 \end{bmatrix} \]
\[ u_{k} = \frac{1}{\lambda_{1} - \lambda_{2}} \begin{bmatrix} \lambda_{1} & \lambda_{2} \\ 1 & 1 \end{bmatrix} \begin{bmatrix} \lambda_{1}^{k} & 0 \\ 0 & \lambda_{2}^{k} \end{bmatrix} \begin{bmatrix} 1 \\ -1 \end{bmatrix} \]
\[ u_{k} = \frac{1}{\lambda_{1} - \lambda_{2}} \begin{bmatrix} \lambda_{1} & \lambda_{2} \\ 1 & 1 \end{bmatrix} \begin{bmatrix} \lambda_{1}^{k} \\ -\lambda_{2}^{k} \end{bmatrix} \]
If our eigenvalues were rational, I might just solve this by substitution and matrix multiplication. Instead, I'll use Python to solve for \(u_{k}\):
import numpy as np
def fibonacci(k):
lambda1 = (1 + np.sqrt(5)) / 2
lambda2 = (1 - np.sqrt(5)) / 2
X = np.array(
[
[lambda1, lambda2],
[1, 1 ],
]
)
v = np.array(
[
[lambda1**k],
[lambda2**k]
]
)
u_k = (1 / (lambda1 - lambda2)) * (X@v)
F_k = u_k[1][0]
return int(round(F_k))
for i in range(1, 10):
print(fibonacci(i))
The output shows the first 9 Fibonacci numbers are \([0, 1, 2, 3, 5, 8, 13, 21, 34]\)
After all that effort you might have been successful in calculating \(F_{100}\) manually, but now we can do it immediately by first calculating \(u_{100}\) and taking it's second element to find \(F_{100}\):
\[F_{100} = 354224848179263111168\]